- HubPages»
- Technology»
- Computers & Software»
- Computer Science & Programming»
- Programming Languages
Controlling program flow in C using if and switch
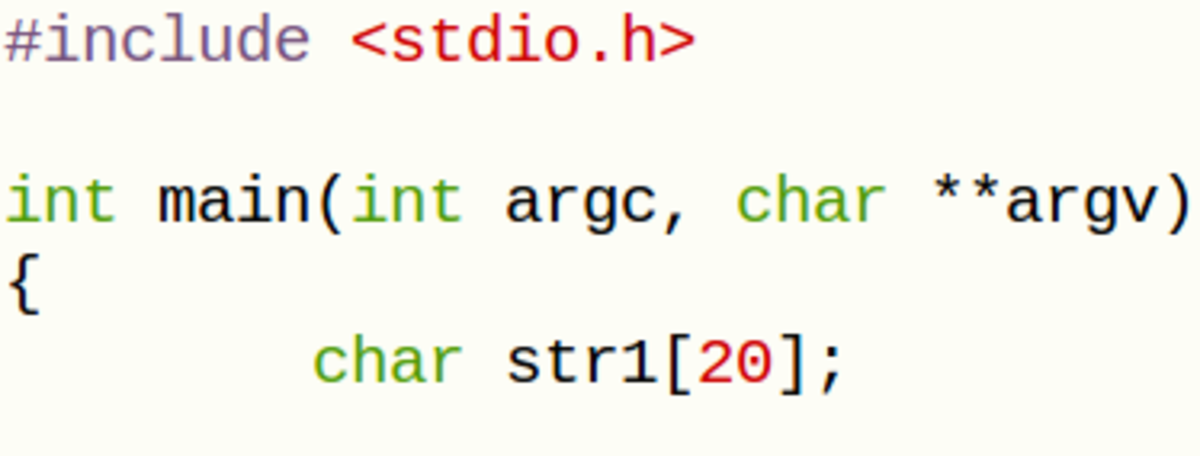
In this hub I'll be showing you how you can execute or not execute statements based on some conditions. We'll be using the keywords "if" and "switch" to achieve this.
This hub is part of a series I'm doing regarding C programming in Linux. If you haven't already, please do check it out so you'd know the full coverage. You'll also find direct links there to the other topics.
Some notes about C statements
To recall, statements in C are any combination of literals, identifiers, keywords and symbols that achieve something. C uses the semi-colon to separate statements, although it functions more like a "terminator" rather than a separator.
Multiple statements can be grouped together using the curly brackets "{" and "}"; this is known as a "statement block".
The statements in a C program are executed from top to bottom. You can also put multiple statements in one line and in this case, the statements will be executed from left to right.
You'll see below that with the use of conditional statements, you can prevent some statements from being executed under certain conditions.
if statement
The keyword if has the following usage specification ("syntax"):
if ( expression )
statement ;
The statement immediately following the "if statement" will be executed only if the expression in the "if statement" evaluates to a non-zero value. Like for example:
if (2 + 5)
x = x + 12;
The assignment statement "x =" above will be executed since the expression "2 + 5" evaluates to "7" which is not zero. If the expression evaluates to zero, then the statement will not be executed.
If there are more than one statement that you'd like to be affected by the "if statement", then you will need to put the statements into a block. Like this:
if(x > 3) { x *= 3; y = x + 5; }
if-else statement
In addition to the "if statement", you can also use the keyword "else" in order to create an alternative code block to be executed if the expression in the "if statement" is false. Please look at the example below:
if (x > 3) x = x * 2; else x = x * 5;
As before, if you like to effect the "else" statement to multiple statements, you'll need to put them into a block. Like for example the code below:
if (x > 4) x *= 4; else { x *= 3; y = x + 2; z = y * (x + 1); }
if-else-if
Another popular use of the "if statement" is to string or chain them together in order to test various conditions, like the code below:
if (a == 1) y = x * 2; else if (a == 2) y = x * 4; else if (a >= 3) y = x + (b * 5); else y = 0;
The "if-else" statement above will be evaluated starting from the top-most "if" statement. If the expression evaluates to 0 ("false"), then the next "else if" will be evaluated. If still it results to false, the next "else if" will then be evaluated and so on and so forth.
If one of the "if" statements above results to non-zero ("true"), then the statement next to it will be performed and all the other "else if" statements are then skipped.
In other words, looking at our example above, if the first "if statement evaluates to false, then let's say the second "else if" ("else if (a == 2)") evaluates to a non-zero value, which means "true", the statement "y = x * 4" will be performed then the next "else if" and the "else" statements will not be evaluated at all.
Note that multiple statements inside the "if" or "else" will need the curly brackets as described above.
switch statement
The "switch" statement is used to check for equality. It can only do integer comparison and doesn't support ranges like "less than" or "greater than". It is used in conjunction with the keyword "case".
The "switch-case" statement block has the following syntax:
switch (expression) { case constant-expression: statements; default: statements; }
The expression in the switch statement will need to yield an integer value. This value is then compared against the value for each of the case statement starting from top to bottom. If a match is found, code execution will begin from there.
It's important to note that the "case" statements only denote where to begin execution given a match. This does not mean that if a match is found in one of the "cases" that the other cases are ignored. To illustrate this, please consider the switch-case statement block below:
switch (a) { case 1: y = x * 2; case 2: y = x * 4; case 3: y = x + (b * 5); default: y = 0; }
The switch statement is testing the expression "a", which is just a variable. In other words, it's testing the value of the variable against the cases below it.
The value next to the "case" keyword is the value tested against the result of the expression in the switch. In other words, "case 1" is like "if ( x == 1 )".
When "a" is equal to the value "1", the first case will match and the code "y = x * 2" will be executed. Now, even though "case 2" did not match, execution will continue and the code "y = x * 4" will also be executed. This behavior is almost always not what you want but can also be useful at times.
break keyword
To prevent the "fall-through" effect we've seen above wherein statements under "case 2" and downwards are executed even though only "case 1" matches, we will use the keyword "break".
When "break" is encountered, execution immediately jumps to the next statement "after" the switch block. So we'll change the switch block above and incorporate some "breaks".
switch (a) { case 1: y = x * 2; break; case 2: y = x * 4; break; case 3: y = x + (b * 5); break; default: y = 0; break; }
With the "breaks" added above, only the statements inside the "case" that matches will be executed. This makes it behave similar to the "if-else-if" chain we've seen above.
Note that you don't need curly brackets for the statements for each "case" since technically, the "case" statements are really "labels" (Note the use of colons ":" at the end of a case statement.). The statements inside the "switch" will need to be enclosed with the curly brackets, however, as you can see above.
Finally, there's the "default" case. Sure enough, by the sound of it, this represents a case that will be executed if none of the cases match. Normally this "default" case is placed after the last "case" and being at the bottom, does not require a "break". However, it is advisable that you do put a "break" for the "default" case so that you can move it up quite easily. Also it allows you to easily add a case after it if you need to.
I highly encourage you to experiment with these keywords as they're almost always used in any program. Try re-arranging stuff or breaking the syntax and see what happens. Try using semi-colons instead of colons after the "case" labels and see what happens. It's really helpful to familiarize yourself with the error or warning messages that the compiler spits out. This can save you a lot of time when tracing your program when things go wrong.