- HubPages»
- Technology»
- Computers & Software»
- Computer Science & Programming
While Loop Example in C Programming Language
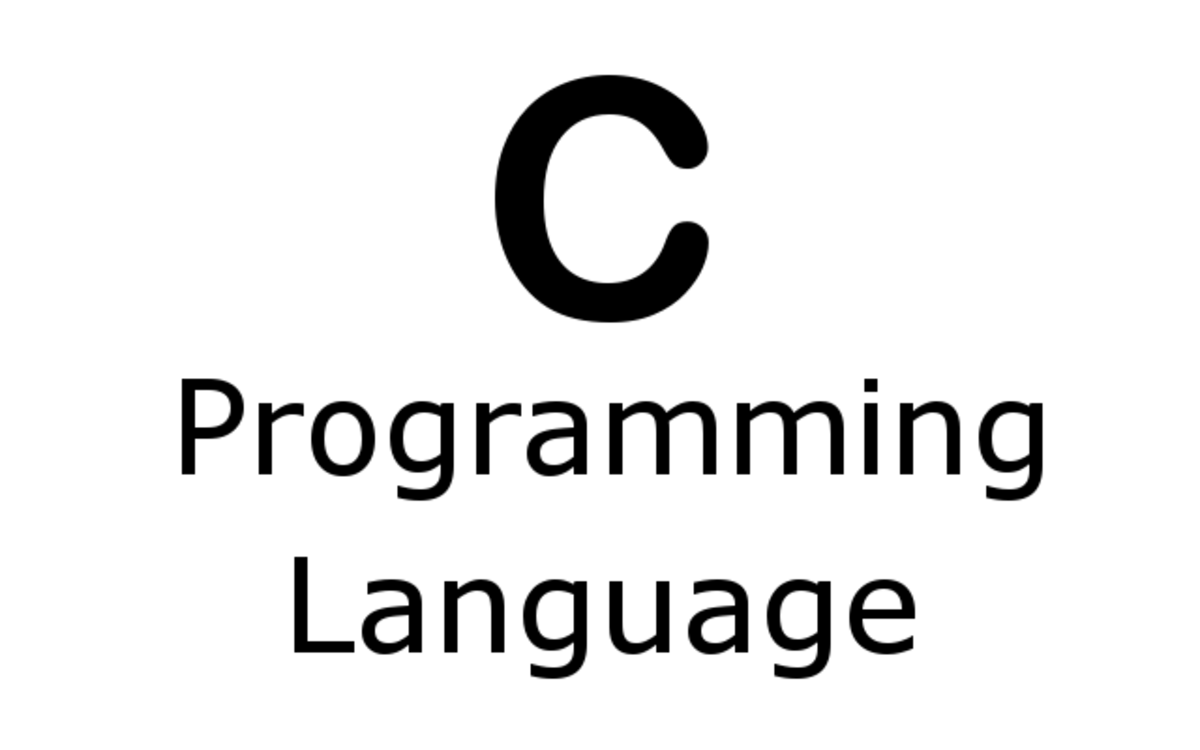
I already explained what is loop and why we use loop in c language, and I am not going to repeat that here again. So we will start with while loop explanation.
While loop is one of the simple loop available in C language.It’s very easy to use, lets check its syntax first and then one simple program.
while loop syntax
initialize loop variable; while(condition) { statement 1; statement 2; statement 3; …………………. statement N; increment loop variable; }
In order to use while loop in any program we need to have one variable which needs to be initialized. Then we can use that variable in while loop, initialization of the variable is one of the important steps in loop usage. I’ll tell you later why it is important. Next in while loop bracket we have to provide condition for loop execution. Condition basically instruct loop on what condition loop will be executed or in simple word how many time repeat the statement written inside loop. As you can notice I have written last line as “increment loop variable”, this is also one of the important step in loop usage, why I am coming on that on “Points to remember” section of this article.
Let’s take look at simplest example of While loop. Below is the example code, it only prints “while loop” text with line number, see the output for better understanding.
while loop example
#include<stdio.h> #include<conio.h> void main() { int i=1; clrscr(); while(i<=10) { printf("%d : while loop.\n", i); i++; } getch(); }
while loop code explanation
In this simple while loop example, we need to concentrate on line no. 10 to 14 and I think with rest of code you are well versed.
While loop begins with “while” keyword as you guys know and in bracket you have to provide condition. In case of my program, condition is “i<=10”, which tells compiler to repeat the execution of code till value of “i” is less than or equal to “10”. But did you notice line no. 7, it’s very important as I told you earlier, here I am initializing variable “i”. Now it will contain 1 and our loop will begin from 1. In line no.-12, we are simply printing “while loop” with number message on screen. In the very next line we are incrementing value of “i” by 1.
So, this is our while loop and its usage. I know very basic thing but this tutorial I wrote for beginners only.
Point to remember
1. Always initialize loop counter variable or your loop may not execute in expected behavior. Let take a look of the same program where I am not initializing variable “i”.One more line, line no. 10, I added to show the initial or default value (also known as garbage value).
uninitialized while loop variable example
#include<stdio.h> #include<conio.h> void main() { int i; clrscr(); printf("Value of uninitialized i : %d", i); while(i<=10) { printf("%d : while loop.\n", i); i++; } getch(); }
On my PC, garbage value of "i" is 886 and could be different on your PC. Now for a moment think, if “i” has value 886, will it ever execute our loop block? We are telling compiler to execute loop block only if value of “i” is less than or equal to 10 (i<=10). I think, now it’s clear why loop counter variable initialization is important. Isn’t it?
2. Never forget to increment loop counter variable inside loop. Due to any reason if you forgot to increment loop counter then your program will stuck in indefinite loop (endless loop). To demonstrate this indefinite loop we will use the same example but we will comment line no. 13. So now you program will look like below one.
infinite while loop example
#include<stdio.h> #include<conio.h> void main() { int i; clrscr(); printf("Value of uninitialized i : %d", i); while(i<=10) { printf("%d : while loop.\n", i); //i++; } getch(); }
Did you notice output? Precisely the line no, it’s always printing 1. Let me explain you the reason why it stuck in endless loop. Remember the while loop condition part (line no. 10) where we are instructing compiler to execute that loop till value of “i” is less than equal to 10. Now when our program entered into loop block its (value of “i”) value is not increasing and every time while loop condition remains true.
While loop in C Poll
Did this help you to learn While Loop in C?
© 2011 RAJKISHOR SAHU